Mappedin Web is a fully packaged solution that can be easily embedded into your website. But what if you wish to include an indoor map in your Flutter app? Mappedin Web can also be used for this purpose. In this blog post we’ll explore how you can do just that.
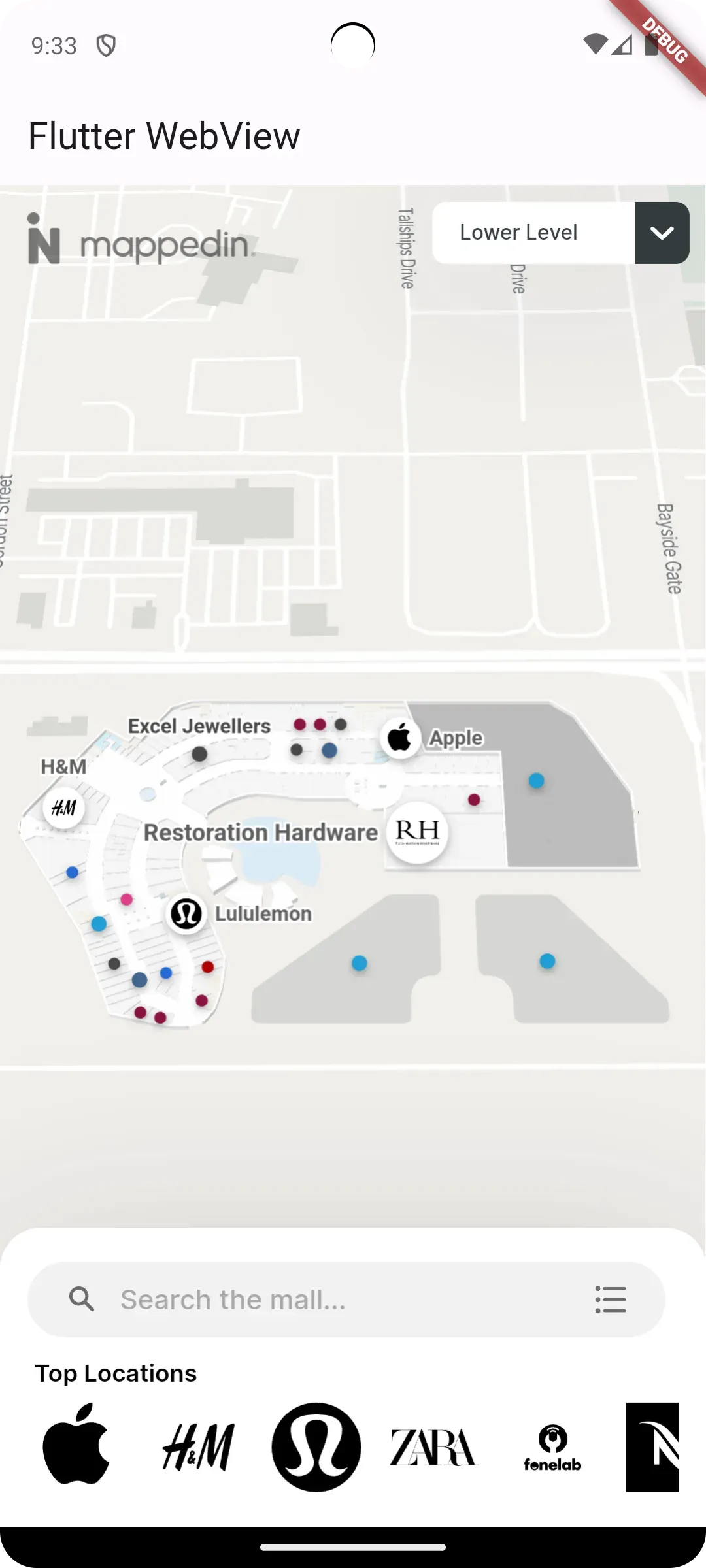
Integrating Mappedin Web into Flutter
Flutter, known for its cross-platform capabilities and ease of use, combined with Mappedin's innovative mapping technology, can bring a whole new dimension to your app. With Mappedin Web, you can provide users with an interactive and engaging map experience. These two solutions can be brought together by using the powerful Flutter InAppWebView.
Code sample
The first step to integrating with Mappedin Web is to create an assets folder within your Flutter project. Within that folder create a new HTML file. For this example, I called it mappedin.html
. Its contents will look like this. Be sure to modify the file to include your own client Id, secret and venue.
Next open the pubspec.yaml
file for your project and add mappedin.html
as an asset under flutter.
Now we are ready to load the HTML file into our app. The sample code below demonstrates how to read mappedin.html
from the app's assets and display it using the flutter_inappwebview
package:
By using the flutter_inappwebview
package, you can easily load your Mappedin Web URL within an InAppWebView widget. This enables your Flutter app users to interact seamlessly with the map while staying within the confines of your application.
Customization with the Mappedin Web SDK
The code snippet above shows how to include the pre-built Mappedin Web into your Flutter app. But what if you desire a more custom solution? The Mappedin Web SDK could also be used in a similar way. You could create a web page using the Mappedin Web SDK that satisfies the use case you desire and embed that page into your Flutter app using Flutter InAppWebView.
Wrapping up
Combining Flutter's versatility with InAppWebView allows you to effortlessly embed Mappedin Web into your app. The provided code snippet serves as a starting point for integrating Mappedin Web, which you can extend to meet your app’s requirements. I hope this made it easy for you to provide users of your Flutter app with indoor maps.
Tagged In
Share