Mappedin’s editing tools, applications, and SDKs make it easy to define and leverage a venue’s spatial information for powerful location-based experiences. For venues like grocery stores, where shelves are typically defined as venue geometry with location, the products that sit on those shelves are typically managed in an external system. Mappedin makes it easy to integrate this external product data with our SDKs in order to power product-based location and navigation experiences.
To demonstrate this integration, we’ll create a web app that leverages Mappedin’s Web SDK to provide product search by name and the walking path to a selected product in a grocery store. If you haven’t yet started with Mappedin’s Web SDK, read our Getting Started Guide on our Developer Portal which introduces the basics of rendering a map view. The following CodeSandbox preview demonstrates this integration using a sample product data set. The full source code for this demo is available here.
In order to associate a product with a location, a relationship needs to be established between the product record in the external system and a location in Mappedin’s Content Management System (CMS).
Defining the product location relationship
In our example, a shelf is defined on the map with visual geometry and a location. That location can store an external identifier (externalId), which can be set to the shelf identifier. The sample venue shown here doesn’t have externalIds defined, so we’ll use polygon names as shelf identifiers. In the external product database, the product record needs to store the associated shelf identifier in order to define the relationship between the product and its current shelf location.
A sample product definition in typescript could look like this:
Products can then be fetched from the external system and integrated into the search capabilities of the SDK.
Integrating products into the SDK search
Customizing the SDK’s offline search feature starts by creating an OfflineSearch object.
For each product fetched from the external system, the search object can be enhanced to support a custom query that defines the query string that users will search for along with the object that should be returned as a result. In this case, the product name is used as the query string and the product object is returned from the search.
Now the search feature has been customized to support searching for products using data coming from an external source.
Displaying search results
To display the product query results as list items, the results can be filtered to only show the items that have custom objects that we added above.
Then, we can create list elements based on the product data:
Adding interaction to these search results will allow us to display a walking path to the product.
Directions to a product
In response to clicking on a search result list item, a Mappedin Journey can be created from the store’s main entrance to the product. This is where the original product and shelf relationship is important - the destination location can be found by looking for the externalId
that matches the product shelfId
.
When clicking on a search result, the map is updated with the new Journey:
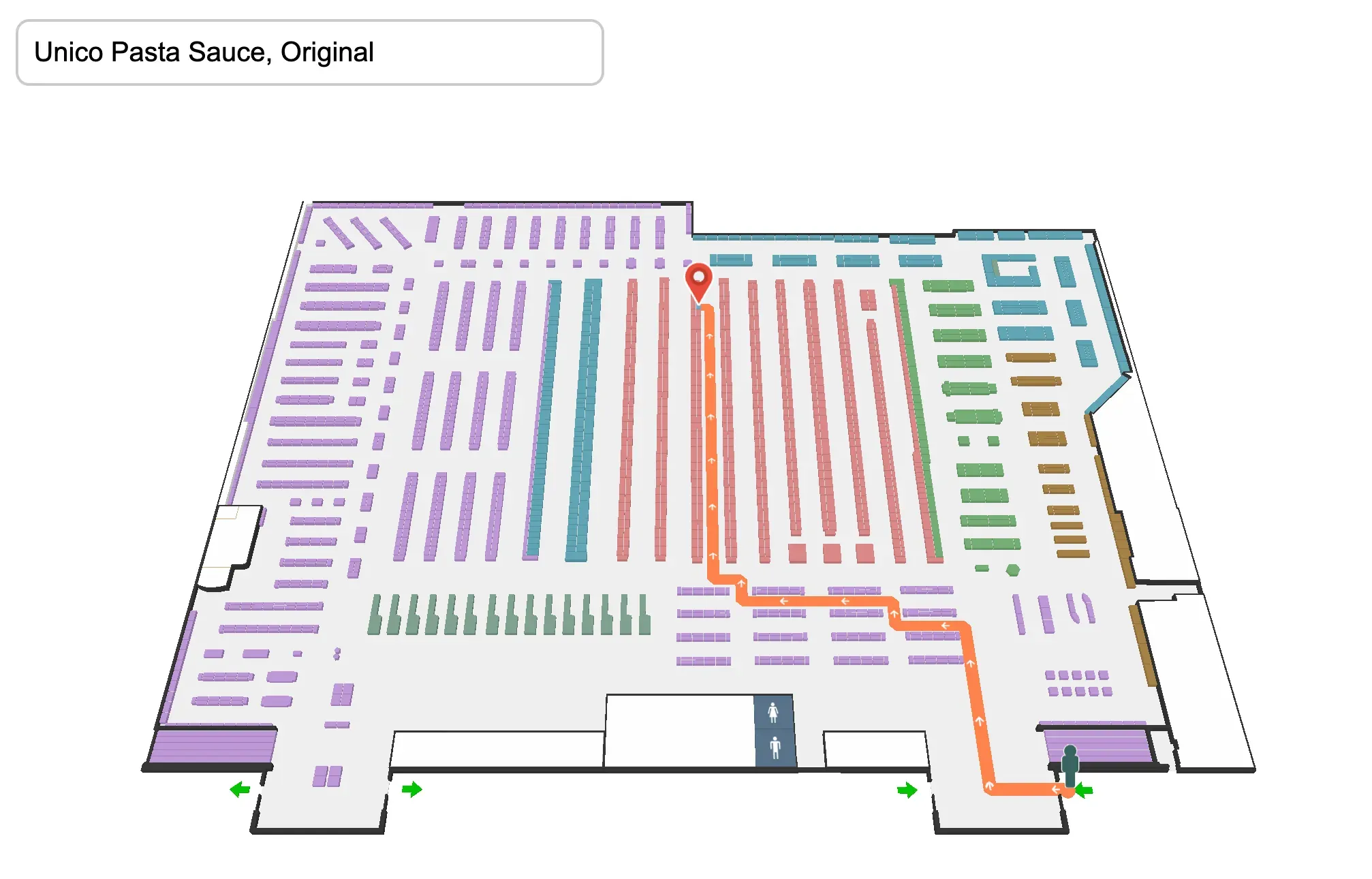
Now users can be guided to products that are stored and managed in an external system. This opens up numerous use cases across various sectors. Retail shoppers can enjoy a streamlined experience, warehouses can optimize inventory management, and large public venues, like museums, libraries, and hospitals, can enhance their visitor and staff experiences with a customized search.
Explore our guides to learn more about Offline Search and Wayfinding, and many more features in our Developer Portal.
Tagged In
Share